Next.js + Kubernetes
Microservices with Node JS and React
The project contains a submodule, clone with these parameters:
git clone –recursive https://github.com/josecho/MicroServicesNatsStreamingBlog.git
The code has changed, to see the code we mentioned in the post:
git checkout handlingPayments
git clone https://github.com/josecho/MSNatsStreamCommon-.git
What is Client-side rendering (CSR)?
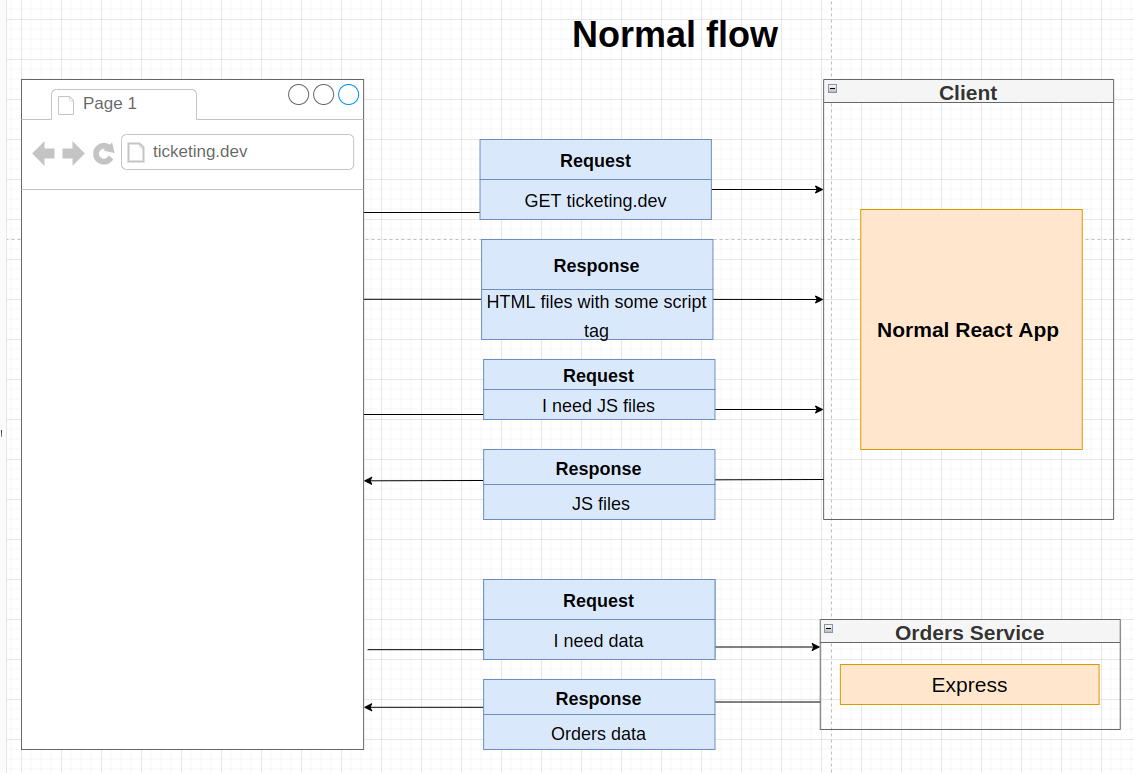
What is server-side rendering?
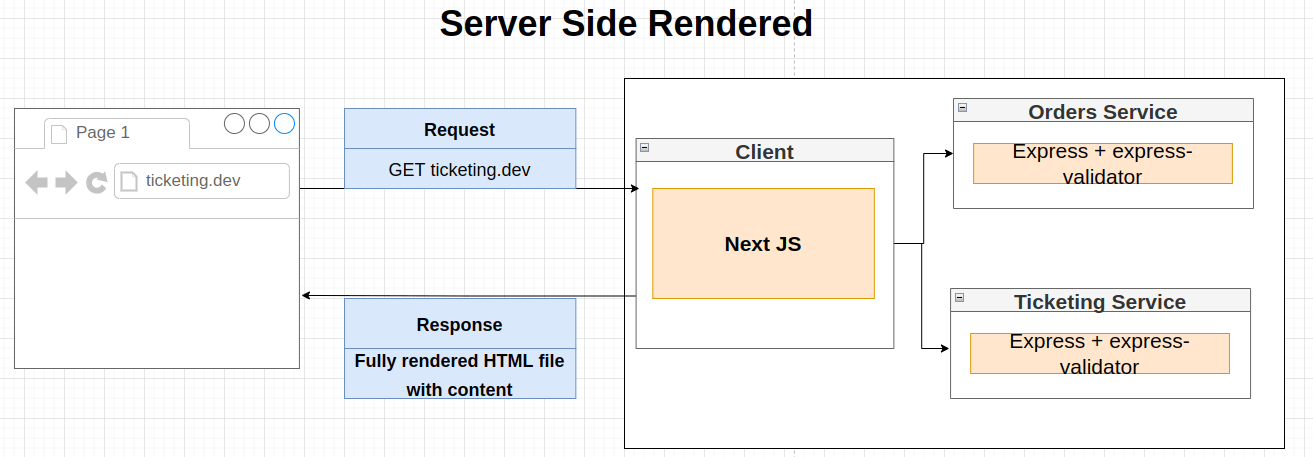
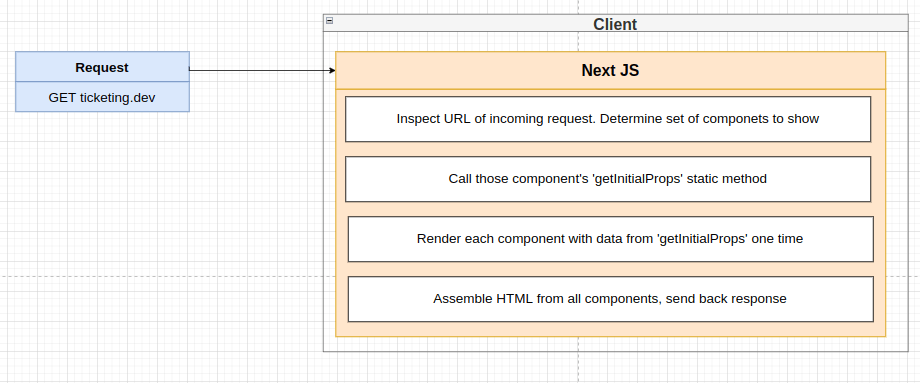
What is Next.js?
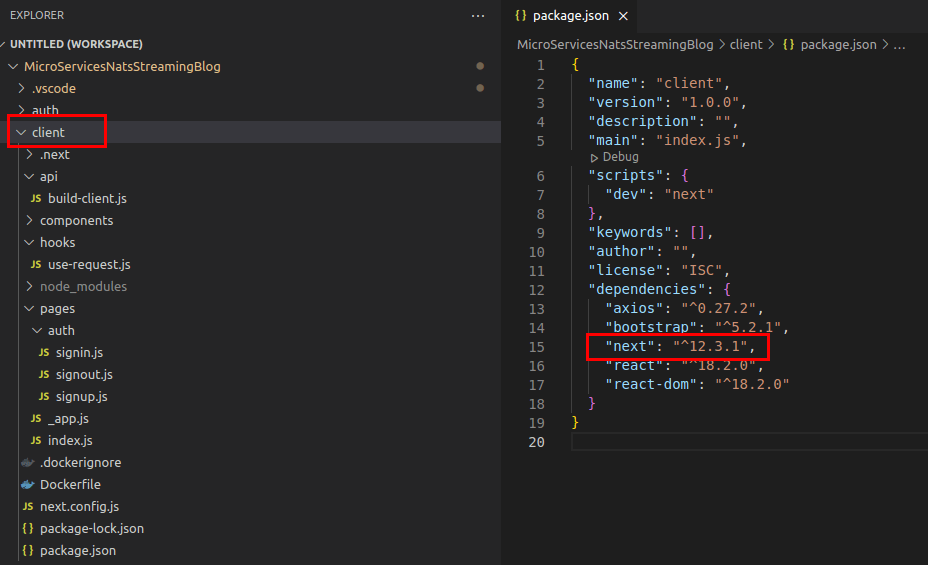
Next.js + Kubernetes
Custom Webpack Config: Next.js + Docker
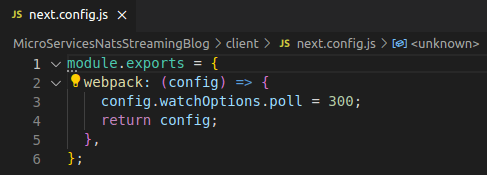
This file is loaded by Next.js every time our project starts. A middleware function modifies the behavior of webpack . Instead of following the default setting to watch for file changes, it is prompted to extract changed files from our project directory every 300 milliseconds.
What is _APP js file in NextJS?
Use _app. js to extend react applications in Next.js. When using Next.js you’ll most likely need to override the global App component to get access to some features like persisting state or global layouts.
Next.js uses the App component to initialize pages. You can override it and control the page initialization and:
- Persist layouts between page changes
- Keeping state when navigating pages
- Custom error handling using
componentDidCatch
- Inject additional data into pages
- Add global CSS
To override the default App, create _app.js file as shown below:
As you can see above, we are customizing the app by adding CSS and creating a header globally. At the same time, AppComponent is wrapping all app components.
So, unfortunately, when we tie getInitialProps to the AppComponent, the getInitialProps function that we tie to an individual page does not get invoked anymore.
For this reason, we must make the call to getInitialProps functions that we tie to an individual page from the App component, as seen in line 20.
if (appContext.Component.getInitialProps) { pageProps = awaitappContext.Component.getInitialProps(appContext.ctx); }
Leave a Reply
Want to join the discussion?Feel free to contribute!